--- layout: presentation title: Lab 2 Slides description: Lab project--Doodle-- --- # CSE 340 Lab 2 (Winter 2020) ## Week 2: Get Help with Doodle .title-slide-logo[ 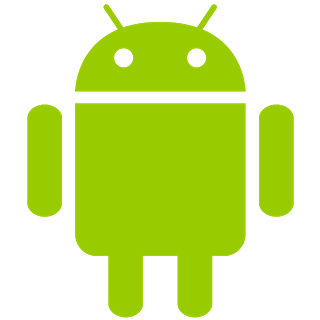 ] --- # Course Objectives - Practical applications for learnings in lecture - Understand design considerations when making mobile apps - Design and implement basic Android applications - Debugging in Java and for Android --- # Timeline - Doodle Due: Thursday, Jan 16 (Today!) - Lock: Saturday, Jan 18 (If you are using late days) - Peer Eval: Sunday, January 19 - Tuesday, January 21 @ 1:30pm - Reflection: Wednesday Jan 22 --- # Lab 2 Objectives - Go through peer evaluation and reflection - How to debug in Andriod - Andriod Animation --- # Lab 2 After Implementation - Peer evaluation - fill the survey that we send out - The best ones will show off in class - Part 3: Turn in reflection on Gradescope after peer evaluation --- # Peer Evaluation - Why? - Chance to externalize your work - Get user feedback (the user experience is after all the point of HCI :)) - What to expect - Your grade won't be determined by peer evaluating alone - You get credit for **doing** the peer evaluation (4 points) - Questions about spec requirements -- no room for interpretation - Questions that prompt about subjective feedback - Provide constructive feedback - Anonymous to your peers (but not to the teaching staff) - Your feedback on the exercise! (be honest) --- # Peer Evaluation Practice - Download the apk file: https://tinyurl.com/wdgjkcu - Google form: https://tinyurl.com/raoqnu2 --- # Peer Evaluation Instructions - You will be assigned to peer grade 4 assignments - If you have an Android phone - Click on the download link to install the app on your phone - If you don't have a phone - Download the APK files - Open the APK file in Android Studio and run the app in the emulator - Fill out the google form for each APK file <img src="./img/wizard.png" alt="screenshot of Android Studio Wizard" width="40%" height="50%"/> --- # Android Debugging - Select a device to debug your app on. - Set breakpoints in your Java, Kotlin, and C/C++ code. - Examine variables and evaluate expressions at runtime - How to use Debugger: https://developer.android.com/studio/debug#java - Helpful tool (Logcat): https://developer.android.com/studio/debug/am-logcat#java --- # Animation on Android - _Property Animation_ - preferred method; more flexible - _View Animation_ - simple setup; the old way (but you have to deal with xml...) - _Drawable Animation_ - load `Drawable` resources and display them one frame after the other (it is like a gif) --- # Property Animation - Define an animate that changes on object's _property_ (a field in an object) over _a lenght of time_ ## `ValueAnimator` - Keeps track of the animation's timing (how long its been running and current value of property) ```java ValueAnimator animation = ValueAnimator.ofFloat(0f, 1f); animation.setDuration(1000); // Starts the animation animation.start(); // TODO: need to listen for updates to get the returned value ``` --- # `ObjectAnimator` (the one you need in this assignment) - Rather than listening for a value, we can simply directly animate a property on an object - **However**: the property that you are animating must have a setter function (in camel case) in the form of set<propertyName>() for this to work (_setDuration()_) ```java ObjectAnimator anim = ObjectAnimator.ofFloat(foo, "alpha", 0f, 1f); anim.setDuration(1000); anim.start(); ``` --- # Resources - Vogella Tutorials - http://www.vogella.com/tutorials/AndroidAnimation/article.html - Android Developer - View Animation - https://developer.android.com/guide/topics/graphics/view-animation.html - Property Animation - https://developer.android.com/guide/topics/graphics/prop-animation.html - Relevant supplemental material is provided on the course website - Listed in the Doodle assignment - Don't stress out :) this isn't required